Batch Processing
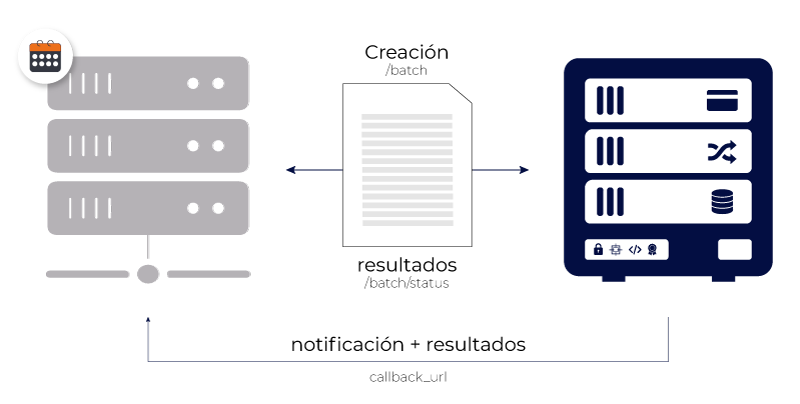
If the Merchant already has a scheduler and only needs to send multiple orders, they can use this batch call to send several charges in a single API call.
Once again, the essential requirement is that the payment data has been tokenized beforehand.
Note that this is an asynchronous call. Our API will receive the batch containing all operations to be processed, and this initial call will only return the status of the file reception. In other words, in this first call identified as (POST /batch)
, the processing result will not be sent.
The mechanisms provided to manage processing results are as follows:
- Callback: In the batch creation call, the
callback_url
parameter specifies a URL in the merchant’s system where the completion notification of the batch and its results will be sent. - On-demand result query: We have enabled an API so that you can check the processing status of a batch. In the creation call, you will receive a
batch_id
parameter, which should be used to check its status.
Batch Authorizations
(POST /batch)
Payload
- operations (array, required): List of all payments to be processed
- amount (string, required): Amount of the operation
1000
- currency (string, required): Any currency defined according to ISO_4217 is valid
EUR
- method(object, required): Contains the necessary data to make the payment.
- type(string, required): Payment method type can only be
sipay
,paypal
, ormovistar_pay
- token(string, required): Identifier with which to make the payment; for
sipay
, it is the token of the stored card, while forpaypal
andmovistar_pay
, they are the billing_id and auth_token respectively (obtained in alternative payments) - extra(object): Extra data for the operation; for movistar_pay, the client_ip_address attribute is mandatory.
- type(string, required): Payment method type can only be
- order (string): Ticket for the operation
sipay-order-001
- reason (string): Available reasons for MIT:
Parameter Reason Description R
Recurring Transactions processed at fixed and regular intervals not exceeding one year between transactions, representing an agreement between the cardholder and a merchant to acquire goods or services provided over a period of time. I
Installments Installment payments describe the one-time purchase of goods or services billed to the cardholder in multiple transactions over an agreed period of time between the cardholder and the merchant. C
One-time Purchase This is a transaction using a stored credential for a fixed or variable amount that does not occur on a scheduled or regular transaction date, in which the cardholder has consented for the merchant to initiate one or more future transactions not initiated by the cardholder. D
Delayed Charge The delayed charge is commonly used in hotels, cruise lines, and vehicle rental settings to make an additional charge to the account after the original services have been provided. E
Resubmission This occurs when the original purchase is made, but the merchant was unable to obtain authorization at the time the goods or services were provided. H
Reauthorization A reauthorization is a purchase made after the original purchase and may reflect a set of specific conditions. Common scenarios include delayed or split shipments and extended stays or rentals. M
Incremental In hotel and car rental environments, there is often an incremental authorization, where the cardholder has agreed to pay for any services rendered during the duration of the contract. N
No Show A "No-show" is a transaction where the merchant is allowed to charge for services that the cardholder had an agreement to purchase but did not fulfill the terms of the agreement. - reference (string): Identifier for bank reconciliation (p37)
1234-sipay
- callback_url (string): URL to receive notification of batch completion.
(optional, recommended.)
- custom_01 (string): Custom field
custom_001
- custom_02 (string): Custom field
custom_002
- amount (string, required): Amount of the operation
Batch Authorization Example
{
"key": "589365da65c48cff87d0874a",
"resource": "359ef8ce5c5f4003b71692e446908c27",
"nonce": "1234567890",
"mode": "sha256",
"payload": {
"callback_url": "https://notify.me/",
"operations": [
{
"amount": "120",
"currency": "EUR",
"order": "order1",
"reason": "R",
"method": {
"token": "2f1897faf4974ea0aac597be71edb061",
"type": "sipay"
},
"reference": "1234"
},
{
"amount": "100",
"currency": "EUR",
"order": "order2",
"reason": "I",
"method": {
"token": "B-70J394298W4258120",
"type": "paypal"
},
"reference": "1234"
},
{
"amount": "5",
"order": "order3",
"reason": "M",
"currency": "EUR",
"method": {
"token": "84ff6564-5001-4825-818c-05bf032cd0d1",
"type": "movistar_pay",
"extra": {
"client_ip_address": "127.0.0.1"
}
},
"reference": "1234"
}
]
}
}
Success - 0 - batch
- batch_id (string): Identifier of the created batch, e.g.,
5a796ad3fba05b3e6ef244e6
Example
{
"type": "success",
"code": "0",
"detail": "batch",
"description": "Batch saved successfully",
"uuid": "2df61337-c0c5-4b90-ab8c-6098c8b118ce",
"request_id": "5901ba6d7710014a2c89",
"payload": {
"batch_id": "5a796ad3fba05b3e6ef244e6"
}
}
Example of Notification Received at callback_url
- Method: POST
- Header included: Content-Type: application/json
{
"transactions": [
{
"status": "completed",
"code": 0,
"reference": "1234asdf",
"order": "order1",
"currency": "EUR",
"custom_01": "",
"custom_02": "",
"amount": 120,
"country": "ES",
"method": "sipay",
"detail": "OPERACIÓN ACEPTADA",
"country_code": 724
},
{
"status": "completed",
"code": 0,
"reference": "1234asdf",
"order": "order2",
"currency": "EUR",
"custom_01": "",
"custom_02": "",
"amount": 50,
"country": "ES",
"method": "sipay",
"detail": "OPERACIÓN ACEPTADA",
"country_code": 724
}
],
"status": "completed",
"records": {
"total": 2,
"success": 2,
"errors": 0
},
"batch_id": "5bbcc11702e2d048ce3a62e7"
}
Batch Query
(POST /batch/status)
Payload
- batch_id (string): Identifier of the created batch, e.g.,
5a796ad3fba05b3e6ef244e6
Batch Query Example
{
"key": "589365da65c48cff87d0874a",
"resource": "359ef8ce5c5f4003b71692e446908c27",
"nonce": "1234567890",
"mode": "sha256",
"payload": {
"batch_id": "5a796be5fba05b3e6ef244e7"
}
}
Success - 0 - card
- batch_id (string): Identifier of the created batch, e.g.,
5a796ad3fba05b3e6ef244e6
- status (string): Status of the batch, e.g.,
completed
created
The batch has been saved and is queued for processingin_process
Payments in this batch are being processedcompleted
All payments in this batch have been processed
- records (object): Object containing a summary of the transaction results.
- total (integer): Total number of operations in the batch, e.g.,
3
- errors (integer): Number of failed transactions, e.g.,
1
- success (integer): Number of successful transactions, e.g.,
2
- total (integer): Total number of operations in the batch, e.g.,
- transactions (array): List of all payments to be processed
- amount (string): Transaction amount, e.g.,
1000
- currency (string): Currency in which the payment was processed, e.g.,
EUR
- status (string): Transaction status, e.g.,
failed
created
Payment is queued for processingin_process
Payment is being processedcompleted
Payment was successfully completedfailed
Payment failedretrying
Payment could not reach the service and is being retried
- method (object): Contains the necessary data to perform the payment.
- type (string): Payment method type, only
sipay
,paypal
, ormovistar_pay
- token (string): Identifier used to make the payment; for
sipay
it's the stored card token
- type (string): Payment method type, only
- order (string): Transaction order ticket, e.g.,
sipay-order-001
- reference (string): Transaction reference; for sipay, this is also the identifier for bank reconciliation (p37), e.g.,
1234-sipay
- custom_01 (string): Customizable field, e.g.,
custom_001
- custom_02 (string): Customizable field, e.g.,
custom_002
- amount (string): Transaction amount, e.g.,
Example - Success
{
"type": "success",
"detail": "batch_status",
"request_id": null,
"uuid": "aef3ddb8-81b2-4579-924c-f3d3939f76a3",
"code": "0",
"payload": {
"status": "completed",
"records": {
"total": 3,
"errors": 0,
"success": 3
},
"callback_url": null,
"transactions": [
{
"order": "41891c47db22426d9ae93faaa6e26f86",
"custom_01": "",
"currency": "EUR",
"detail": "OPERATION ACCEPTED",
"country_code": 724,
"country": "ES",
"amount": 120,
"reference": "1234",
"custom_02": "",
"code": 0,
"method": {
"type": "sipay",
"token": "2f1897faf4974ea0aac597be71edb061",
"extra": null
},
"status": "completed"
},
{
"order": "48d0b70d51c34a1eb70e83bd8cb16a1d",
"custom_01": "",
"currency": "EUR",
"detail": "operation_succeeded",
"country_code": 724,
"country": "ES",
"amount": 100,
"reference": "1234",
"custom_02": "",
"code": 0,
"method": {
"type": "paypal",
"token": "B-70J394298W4258127",
"extra": null
},
"status": "completed"
},
{
"order": "4d4cdac2b5d44a3aa15bf4586ffc6e65",
"custom_01": "",
"currency": "EUR",
"detail": "Request done successfully",
"country_code": null,
"country": null,
"amount": 5,
"reference": "1234",
"custom_02": "",
"code": 0,
"method": {
"type": "movistar_pay",
"token": "84ff6564-5001-4825-818c-05bf032cd0d1",
"extra": {
"client_ip_address": "127.0.0.1"
}
},
"status": "completed"
}
],
"notify": false,
"batch_id": "5abbaf53cd36c40001d0943c"
},
"description": "Query completed successfully"
}