Accept Charges
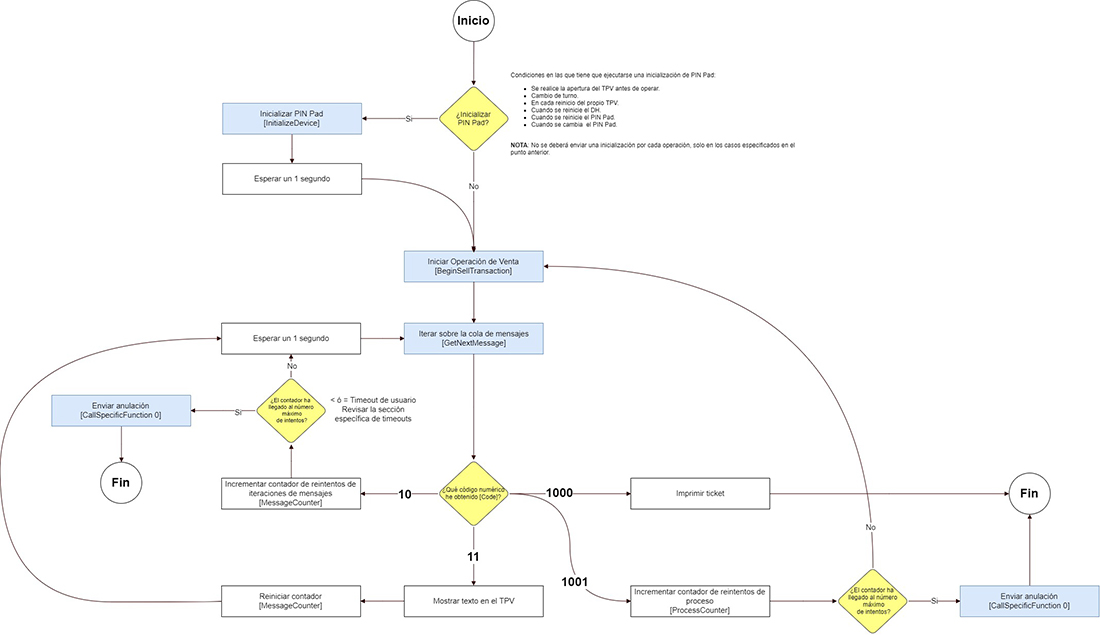
To start processing charges with our in-person solution, you must have a PIN Pad device and a correct installation of DeviceHub with the corresponding configuration. Programmatically, you must integrate at least these three XML messages:
- InitializeDevice: allows the device to be initialized.
- GetNextMessage: iterates through the messages in the device queue and processing.
- BeginSellTransaction: sends a charge order to the PIN Pad.
You should be aware that the integration is asynchronous, meaning, when sending an XML call, normally the DeviceHub will respond if it has received the call correctly but will not respond with the result of an operation. The only exception is the GetNextMessage call, which is precisely intended to go through the messages of the asynchronous queue.
API calls will be HTTP POST requests to the TCP/IP address where the DeviceHub is installed. Normally, this address will be:
POST http://localhost:17000
The structure of the exchanged XML messages has common data, both in the request and in the response. Below we include some generic message prototypes.
Generic Request
In this case, we have chosen the fictitious name of FunctionCallRequest to prototype a call that helps with the explanation.
- ClientIdValue: a numeric identifier provided by Sipay that identifies the client
- StoreIdValue: a numeric identifier provided by Sipay that identifies the merchant
- PosIdValue: an alphanumeric identifier of the point of sale and configurable by the Commerce.
- Language: code of the integration messaging language. 0 is Spanish.
<?xml version="1.0" encoding="UTF-8"?>
<soap:Envelope xmlns:soap="http://www.w3.org/2003/05/soap-envelope" xmlns:tem="http://tempuri.org/">
<soap:Header/>
<soap:Body>
<tem:FunctionCallRequest>
<tem:Header>
<tem:ClientId>ClientIdValue</tem:ClientId>
<tem:StoreId>StoreIdValue</tem:StoreId>
<tem:PosId>PosIdValue</tem:PosId>
<tem:Lang>Language</tem:Lang>
<tem:ExtraData1></tem:ExtraData1>
</tem:Header>
</tem:FunctionCallRequest>
</soap:Body>
</soap:Envelope>
Generic Response
In common response values, we are mainly interested in:
- Code: a code 0 if the call was successful, any other value indicates that there was a problem.
- Description: descriptive literal of the result of the call.
<?xml version="1.0" encoding="UTF-8"?>
<SOAP-ENV:Envelope xmlns:SOAP-ENV="http://www.w3.org/2003/05/soap-envelope"
xmlns:SOAP-ENC="http://www.w3.org/2003/05/soap-encoding" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:ns2="http://tempuri.org/SipayPlusAccess"
xmlns:ns1="http://tempuri.org/">
<SOAP-ENV:Header></SOAP-ENV:Header>
<SOAP-ENV:Body>
<ns1:FunctionCallResponse>
<ns1:Result>
<ns1:Code></ns1:Code>
<ns1:Description></ns1:Description>
</ns1:Result>
</ns1:FunctionCallResponse>
</SOAP-ENV:Body>
</SOAP-ENV:Envelope>
InitializeDevice - Initialize Device
This function is used to initialize the device, set up the local date and time on the PIN Pad, retrieve the device's key version, and register it in the Sipay database, associating it with a client number, store, and point of sale (POS), as well as other administrative parameters.
The gateway only allows one PIN Pad per POS. All merchants must ensure that in the same merchant (StoreID) they do not repeat the POS among the different POS terminals they have. With the daily initialization of the PIN Pad upon opening the cash registers, the serial number linked to a client ID, a store ID, and a POS ID is sent to the gateway. These data are stored in Sipay's payment gateway and when transactions are processed, the PIN Pad serial number travels to Redsys as part of the data to validate and authorize the operation. If the POS is repeated, the sale could be sent with the wrong PIN Pad and cause a WRONG PIN error.
For the synchronization of the date and time, the device hub uses the local system's date/time, so it is very important that the system clock is correct. A date discrepancy could cause a terminal lockout for security reasons, rendering it permanently unusable.
The PIN Pad must be initialized in the following cases:
- When opening the POS terminal before operating.
- When changing shifts.
- At each restart of the POS terminal itself.
- When restarting the DeviceHub.
- When restarting the PIN Pad.
- When changing the PIN Pad.
<soap:Envelope xmlns:soap="http://www.w3.org/2003/05/soap-envelope" xmlns:tem="http://tempuri.org/">
<soap:Header/>
<soap:Body>
<tem:InitializeDeviceRequest>
<tem:Header>
<tem:ClientId>ClientIdValue</tem:ClientId>
<tem:StoreId>StoreIdValue</tem:StoreId>
<tem:PosId>PosIdValue</tem:PosId>
<tem:Lang>Idioma</tem:Lang>
<tem:ExtraData1></tem:ExtraData1>
</tem:Header>
</tem:InitializeDeviceRequest>
</soap:Body>
</soap:Envelope>
IMPORTANT: The call should not be made before each operation, as it can cause a significant delay in the operation of the PIN Pad.
BeginSellTransaction - Request Payment
This function, once an amount and a ticket number have been provided, launches the sales transaction requesting financial authorization. The response code indicates whether the request has been delivered to the DeviceHub service. To obtain the transaction result, the GetNextMessage function must be used.
Uniqueness of the "Operation – Ticket Number" Relationship
It is important that the relationship between a ticket number and a purchase operation by a user is unique. If the gateway receives several different ticket numbers, it will interpret them as different operations.
Thus, for the same sales operation, regardless of the number of times the same is attempted, the ticket number sent from the register must be the same. The purpose of this rule is to avoid duplications in operations.
The gateway considers an operation duplicated if the following parameters coincide:
- Client
- Store
- Ticket Number
- POS
- Amount
IMPORTANT: The card number is not considered, so in the case of a split bill with the same amount, a different ticket must be generated to avoid automatic cancellations by detecting duplications.
<soap:Envelope xmlns:soap="http://www.w3.org/2003/05/soap-envelope" xmlns:tem="http://tempuri.org/">
<soap:Header/>
<soap:Body>
<tem:BeginSellTransactionRequest>
<tem:Header>
<tem:ClientId>ClientIdValue</tem:ClientId>
<tem:StoreId>StoreIdValue</tem:StoreId>
<tem:PosId>ValuePosIdValue</tem:PosId>
<tem:Lang>Idioma</tem:Lang>
<tem:ExtraData1></tem:ExtraData1>
</tem:Header>
<tem:Amount>Importe</tem:Amount>
<tem:TicketNumber>TicketNumber</tem:TicketNumber>
</tem:BeginSellTransactionRequest>
</soap:Body>
</soap:Envelope>
Request: BeginSellTransactionRequest
TYPE | NAME | COMMENTS |
---|---|---|
String | Amount | Sale amount. The transaction amount with 10 numeric digits, where the last two digits represent cents. Ex: 1€ -> 0000000100; 2.25€ -> 0000000225 |
String | TicketNumber | Sale receipt number. |
Response: BeginSellTransactionResponse
<?xml version="1.0" encoding="UTF-8"?>
<SOAP-ENV:Envelope xmlns:SOAP-ENV="http://www.w3.org/2003/05/soap-envelope" xmlns:SOAP-ENC="http://www.w3.org/2003/05/soap-encoding" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:ns2="http://tempuri.org/SipayPlusAccess" xmlns:ns1="http://tempuri.org/">
<SOAP-ENV:Header></SOAP-ENV:Header>
<SOAP-ENV:Body>
<ns1:GetNextMessageResponse>
<ns1:Result>
<ns1:Code>0</ns1:Code>
<ns1:Description>Ok</ns1:Description>
</ns1:Result>
<ns1:Message>
<ns1:MessageCode>1000</ns1:MessageCode>
<ns1:MessageText>OPERACIÓN ACEPTADA</ns1:MessageText>
<ns1:PrintableData>
<ns1:OperationType>Venta</ns1:OperationType>
<ns1:AuthorizationMode>O</ns1:AuthorizationMode>
<ns1:ACRCode>00</ns1:ACRCode>
<ns1:DataInputType></ns1:DataInputType>
<ns1:DataVerificationType>*</ns1:DataVerificationType>
<ns1:FUCCode></ns1:FUCCode>
<ns1:Terminal></ns1:Terminal>
<ns1:HCP>CAIXABANK, S.A.</ns1:HCP>
<ns1:Store></ns1:Store>
<ns1:City></ns1:City>
<ns1:CardHolder></ns1:CardHolder>
<ns1:AID></ns1:AID>
<ns1:DDFName>A0000000032010</ns1:DDFName>
<ns1:AppLabel>VISA</ns1:AppLabel>
<ns1:ContactlessLiteral>1</ns1:ContactlessLiteral>
<ns1:CardNumber>************9262</ns1:CardNumber>
<ns1:SequenceNumber>192088942</ns1:SequenceNumber>
<ns1:TerminalLabel></ns1:TerminalLabel>
<ns1:DateTime>25/11/2022 - 11:33:50</ns1:DateTime>
<ns1:TerminalSequence>0295</ns1:TerminalSequence>
<ns1:AuthorizationNumber>813039</ns1:AuthorizationNumber>
<ns1:Amount>0.01</ns1:Amount>
<ns1:CurrencySimbol>EUR</ns1:CurrencySimbol>
<ns1:InfoText>REDSYS</ns1:InfoText>
<ns1:Aclaratory></ns1:Aclaratory>
<ns1:ExtraData1><ExtraData1><EXTRA_DATA><DCC><EXCHANGE_RATE/><MARK_UP/><COMMISION/><PERCENT_MARGIN_EXCHANGE_BCE/><EXCHANGE_RATE_BCE/><DCC_CONVERSION_FACTOR/><DCC_MERCHANT/><DCC_AMOUNT/><DCC_CURRENCY_CODE/></DCC><COMMERECE><IDENT>SIPAY PLUS S.L.</IDENT><ADDRESS>AVENIDA EUROÇA 14</ADDRESS><TEL>607067689</TEL><STORE>607067689</STORE><CITY>ALCOBENDAS</CITY><CIF>B78990988</CIF><TICKET>srs_redsys</TICKET></COMMERECE><CASHBACK><COMMISSION_TYPE/><COMMISSION/><CURRENCY/><ADDITIONAL_COMMISSION_TYPE/><ADDITIONAL_COMMISSION/></CASHBACK><CARD_INFO><BIN>404700</BIN><FAMILY_ID>20</FAMILY_ID><FAMILY_NAME>VISA</FAMILY_NAME><EMIT_BANK_CODE>2100</EMIT_BANK_CODE><EMIT_BANK_NAME>CAIXABANK, S.A.</EMIT_BANK_NAME><ADQ_BANK_CODE>2100</ADQ_BANK_CODE><ADQ_BANK_NAME>CAIXABANK, S.A.</ADQ_BANK_NAME><BANK_NETWORK>A101</BANK_NETWORK><FUNCTION_ID>2</FUNCTION_ID><FUNCTION_NAME>DEBIT</FUNCTION_NAME></CARD_INFO><PRIVATE_CARD><INFO_TYPE/><REFERENCE/><DESTINY/><LETTER/><PAYMENT_INFO/></PRIVATE_CARD></EXTRA_DATA></ExtraData1></ns1:ExtraData1>
<ns1:ExtraData2></ns1:ExtraData2>
</ns1:PrintableData>
<ns1:ExtraInfo>
<ns1:TaxFreeEnabled>0</ns1:TaxFreeEnabled>
<ns1:Feature1>0</ns1:Feature1>
<ns1:Feature2>0</ns1:Feature2>
<ns1:Feature3>0</ns1:Feature3>
<ns1:Feature4>0</ns1:Feature4>
<ns1:AuthorizationCode></ns1:AuthorizationCode>
<ns1:FeatureInfo></ns1:FeatureInfo>
</ns1:ExtraInfo>
</ns1:Message>
</ns1:GetNextMessageResponse>
</SOAP-ENV:Body>
</SOAP-ENV:Envelope>
TYPE | NAME | COMMENTS |
---|---|---|
Result | Result | Returns 0=OK or an error code and its description. |
Result
TYPE | NAME | COMMENTS |
---|---|---|
Int | Code | Error code. |
String | Description | Error description. |
Ticket Printing
The cash register software should include a series of data for ticket printing. The mandatory and optional fields are as follows:
MANDATORY FIELDS SALES RECEIPT |
---|
Operation type |
Authorization response code (ARC) (only if the operation is carried out with EMV card) |
Assigned merchant F.U.C. code (merchant identification) |
Terminal |
Merchant name |
Merchant city |
Application Identifier (AID) (only if the operation is carried out with EMV card) |
Card number replacing the first 12 digits with '*' |
Application label with the following priority (only if the operation is carried out with EMV card 'ApplicationLabel(T50), 'Terminal Label') |
Date and time of the operation (DDMMAA hhmm) |
Authorization number |
Operation amount with currency indication |
Merchant receipt: Signature box, whenever it is the method applied for cardholder authentication or when not, if not programmed in the terminal. |
Merchant receipt: In case the cardholder authentication is done by PIN and the terminal is not programmed to request the signature, it is recommended to include the text "PIN TRANSACTION. NO SIGNATURE REQUIRED" and the signature box will not be required to print. |
Merchant receipt: It will be indicated that the receipt is for the merchant. |
Customer receipt: Indicate that it is a copy for the customer |
Remaining copies: Indicate that it is a copy |
OPTIONAL FIELDS |
---|
Cardholder (some companies include it and others don't) |
Authorizing center |
Payment gateway |
Important Considerations for Ticket Printing
For Contactless transactions, it is mandatory to print the logo. To determine whether the contactless logo should be printed or not, simply check the ContactlessLiteral variable:
IF (ContactlessLiteral == 1 OR ContactlessLiteral == 2) the logo should be placed, otherwise not.
Regarding the authentication method of the transaction, the texts follow the DataVerificationType variable.
SWITCH (DataVerificationType)
BEGIN
CASE 'P':
text = "PIN TRANSACTION, NO SIGNATURE REQUIRED"
break;
CASE 'F':
text = "SIGNATURE REQUIRED, NO PIN TRANSACTION"
break;
~ CASE 'PF':
text = "PIN AND SIGNATURE TRANSACTION"
break;
CASE '*'
text = "CONTACTLESS TRANSACTION, NO SIGNATURE OR PIN REQUIRED"
END
Based on the received value, the following actions should be taken:
- In cases F and PF, the signature box should be printed on the ticket.
- Regarding chip cards, it is crucial to remember that the following scenarios can occur:
- Request signature (similar to contactless).
- Neither request signature nor PIN without being contactless.
Therefore, it is very important to adhere to the rules provided by the DataVerificationType, as they represent how and under what conditions the entity has authorized the transaction. Failure to comply with the rules renders the ticket invalid in case of a bank repudiation.
The ticket printing will depend on the Pin Pad model used:
- Fixed PIN Pads (integrated): The printing and ticket fields depend on the POS terminal.
- Wireless PIN Pads (standalone): The printing and ticket fields depend on the Sipay gateway.
It is important to note that ticket printing represents the completion of the transaction. If the ticket is not printed (either on the POS terminal or the PIN Pad itself), the transaction will not have been completed, requiring the retry of the transaction using the same ticket number and amount.
GetNextMessage - Traverse Output Messages
Once the transaction has started, whether it's a sale or a refund, this function allows retrieving messages from the device to understand at which step of the process the operation is.
It is important to control the maximum time to receive a code 10 in the flow of the GetNextMessage function. This is a configurable interval set by the client, for which it is recommended that the Timeout be equal to or greater than the User Timeout.
Once this Timeout is reached, the call must be stopped, i.e., stop the request of GetNextMessage, and send a cancellation of the operation with the ticket number. This applies to sale, refund, and preauthorization operations.
It is important to note that if the waiting time is exceeded or there is any error during the transaction, it is very important to call the Cancel function with the ticket number.
After launching the cancellation call, the circuit must be followed to verify with the GetNextMessage function. Getting a 1000 represents that the device hub has received the cancellation request correctly, but this does not indicate that it has been processed at that moment. The cancellation process is asynchronous and will be performed when the device hub has a connection with the entity.
To verify that such operation has been canceled, the Sipay Plus operations website must be checked, considering that this process can take up to 24 hours.
The following table shows a description of the code returned by the GetNextMessage function call.
Código | Comentarios |
---|---|
10 | Signifies that the gateway has no response for the current step of the process, and therefore, it should continue querying and iterating in the message queue (GetNextMessage) to proceed with the process. |
11 | Will be displayed on the POS terminal or Pin Pad for the next step to continue with the operation. For example, you may receive a code 11 when the card is requested or when the PIN or signature is requested. Additionally, whenever you receive a code 11, you must reset the timeout to 2 minutes so that the operation does not stop due to timeout and give the customer enough time to complete each step of the purchase. |
1000 | The operation has been accepted and authorized. Consequently, the sale ticket will be printed, and the process concludes. The printing of the ticket by the POS terminal represents the end of the operation. |
1001 | The payment has not been successful, meaning the payment has not ended successfully either due to technical reasons or because the entity in its response indicates that it cannot continue that operation satisfactorily (for example, denied for various reasons). In this case, the ticket will not be printed, and the operation should be retried as appropriate for your business, always using the same ticket number to ensure that the gateway's consistency checks work properly. |
Response: GetNextMessageRequestResponse
TYPE | NAME | COMMENTS |
---|---|---|
Result | Result | Returns 0=OK or an error code and its description. |
Message | Message | Contains the response code and its corresponding message, as well as storing print information and other extra information for flags. |
Result
TYPE | NAME | COMMENTS |
---|---|---|
Int | Code | Error code. |
String | Description | Error description. |
Message
TYPE | NAME | COMMENTS |
---|---|---|
Int | MessageCode | Transaction response code. |
String | MessageText | Response message. |
PrintableData* | PrintableData | Print data. |
Extrainfo* | Extrainfo | Transaction flags information. |
String* | ExpansionData | Expansion data in XML format. |
PrintableData
TYPE | NAME | COMMENTS |
---|---|---|
String | OperationType | Operation type. |
String | AuthorizationMode | Authorization mode. |
String | ACRCodem | ARC code in EMV transactions. |
String | DataInputType | Data input type in the PIN Pad: C Chip; B Band Reading; M Manual Input |
String | DataVerificationType | Type of verification used to authenticate the buyer. |
String | FUCCode | FUC code, merchant number. |
String | HCP | Authorizing entity/processor. |
String | Store | Not Used. |
String | City | Not Used. |
String | CardHolder | Cardholder, only if traveling in the message. |
String | AID | EMV data. |
String | DDFName | EMV data. |
String | AppLabel | Application used in the PIN Pad. |
String | ContactLessLiteral | Contactless operation indicator. |
String | CardNumber | Masked card number. |
String | SequenceNumber | Transaction sequence number. Can locate a transaction in Sipay Plus. |
String | TerminalLabel | Bank terminal number. |
String | TerminalSequence | |
String | DataTime | Date and time. |
String | AuthorizationNumber | Transaction authorization number. |
String | Amount | Operation amount in the currency in which it was performed. |
String | CurrencySimbol | ISO code, name of the currency in which the operation was performed. |
String | InfoText | Information from the authorizing center. |
String | Aclaratory | Information from the authorizing center. |
String | ExtraData1 | Extra data to print in XML format. See ExtraData1 in the lower table. |
String | ExtraData2 | Future expansion. |
ExtraData1 (XML)
Complementary information is sent according to the type of operation: DCC, private cards, Tax Free, etc. For more detailed information about ExtraData, you can contact our support team.
In ExtraData1, you will find multi-currency operation data (DCC) and merchant data where the operation was carried out. Multi-currency data is mandatory in DCC operation tickets.
In DCC operations, the "Amount" and "CurrencySymbol" fields of PrintableData show the amount and the currency selected during the transaction, so they can always be used. From the DCC subfield of the XML, it will be necessary to extract at least the data "EXCHANGE_RATE", "DCC_MERCHANT", when fed (DCC operations).
<EXTRA_DATA>
<DCC>
<EXCHANGE_RATE/><- Tasa de intercambio aplicada
<MARK_UP/>
<COMMISION/><- Comisión del procesador DCC
<DCC_MERCHANT>CAIXABANK, S.A.</DCC_MERCHANT><- Operador DCC
<DCC_AMOUNT/><- Importe de la operación
<DCC_CURRENCY_CODE/><- Nombre de la moneda
</DCC>
<COMMERECE>
<IDENT>SIPAY PLUS S.L.</IDENT>
<ADDRESS>AVDA. EUROPA 14 PLANTA 1o A</ADDRESS>
<TEL>620857910</TEL>
<STORE>TIENDA SIPAY PLUS 1</STORE>
<CITY>Madrid</CITY>
<CIF>B67890980</CIF>
</COMMERECE>
<CARD_INFO>
<BIN>46456</BIN> !- Bin de la tarjeta que ha operado
<FAMILY_ID>20</FAMILY_ID> !- ID de la familia de la tarjeta (máx 3 dígitos)
<FAMILY_NAME>VISA</FAMILY_NAME> !- Nombre de la familia de la tarjeta (máx 45 dígitos)
<EMIT_BANK_CODE>2100</EMIT_BANK_CODE> !- Código ISO de la entidad emisora de la tarjeta ( 4 dígitos ) <EMIT_BANK_NAME>CAIXA BANK S.A</EMIT_BANK_NAME> !- Nombre de la entidad emisora
<ADQ_BANK_CODE>0049</ADQ_BANK_CODE> !- Código ISO de la entidad adquiriente (4 digitos)
<ADQ_BANK_NAME>BANCO SANTANDER S.A.</ADQ_BANK_NAME> !- Nombre de la entidad adquiriente (máx 45 dígitos)
BANK_NETWORK>A101</BANK_NETWORK> !- Nombre de la red en que opera la tarjeta
</CARD_INFO>
<PRIVATE_CARD>
<INFO_TYPE> tipo de información </INFO_TYPE>
<REFERENCE>nº de referencia de la operación</REFERENCE>,
<DESTINY> destino (impresión o pantalla) </DESTINY>,
<LETTER> tipo de letra </LETTER>,
<PAYMENT_INFO> datos enviados por la entidad sobre el pago</PAYMENT_INFO>
</PRIVATE_CARD>
</EXTRA_DATA>